Variables, Data Types, and Operators in JavaScript
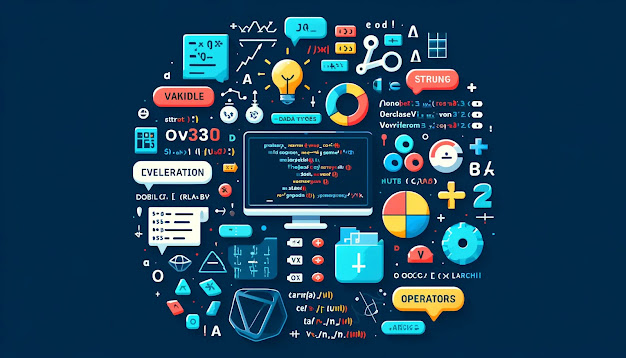
Variables 1. Declaration var Historically, variables were declared using the var keyword. However, it has some scoping issues and is generally replaced by let and const in modern JavaScript. javascript var x = 10; let Introduced in ES6, let allows you to declare block-scoped variables. This means the variable is only available within the block it is defined. javascript let y = 20; const Also introduced in ES6, const is used to declare block-scoped variables that are read-only. Once a value is assigned to a const variable, it cannot be reassigned. javascript const z = 30; 2. Variable Scope Global Scope: Variables declared outside of any function or block are in the global scope and can be accessed from anywhere in the code. Function Scope: Variables declared within a function using var are confined to the function scope. Block Scope: Variables declared with let or const within a block (denoted by {}) are confined to that block. javascript if (true) { var a = 40; // global ...